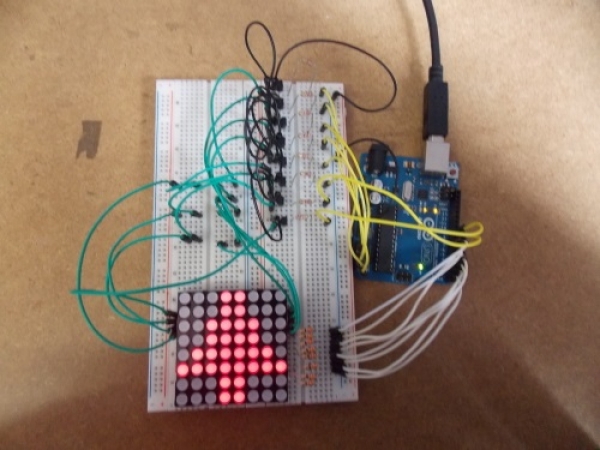
In this Project you will see how to make display a scrolling text message in a led 8x8 matrix with basic components and Arduino. The message can be altered changing the sketch.
Materials List:
1 x Arduino Uno R3
1 x Large Breadboard
8 x 330Ω Resistors (to limit anodo electric current)
8 x 1KΩ Resistors (to uses transistor as switch)
8 x BC337 transistor (a BC547 or any compatible NPN can be used).
1 x Matrix 8x8 LED 2 colors (unicolor also works)
Some Jumpers.
On image below you can see the suggested circuit:
The PORTD (Pins 0 to 7) is connected to 330Ω resistors. The resistor is connected to each display anode.
The PORTC (Pins A0 to A5) and PORTB (Pins 8 and 9) are connected to 1KΩ resistor to each transistor's base. The transistor switch GND to catodo of the LEDs.
How it works:
The program logic is activate one column at a time. The high speed switch between columns cause an effect called Persistence_of_Vision or POV. A picture is completed after 8 columns. For the POV effect we used picture refresh as about 24 frames per second.
Step by step:
The 1st column is activated putting the anode of a column to GND.
The data on matrix text[] is searched using the address indicated by "Pointer" + "Column" variables. This data go to Arduino's PORTD.
A delay for the POV take effect (your eye must retain the image).
The logic repeats the steps above for all columns and the picture is formed.
All above is better explained by the animation bellow:
The picture is repeated some times for speed control.
You will see the letter "A" as below:
After this step, pointer is incremented and program return to first step (scrolling effect ). The limit of array text[] is determined by first for loop logic.
Upload of Sketch:
After checking the assembling, Copy/Paste sketch below and Upload to Arduino:
// // MERRY XMAS Scrolling Text Message //
byte text[] = {//Array of columns used to write on matrix (You can modify text here)
0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0,//Space
0x0, 0x7E, 0xFF, 0xC3, 0xDB, 0xDF, 0x5E, 0x0,//G
0x0, 0xFF, 0xFF, 0x3, 0x3, 0x3, 0x3, 0x0,//L
0x0, 0x18, 0x18, 0x18, 0x18, 0x18, 0x18, 0x0,//-
0x0, 0xFF, 0x60, 0x30, 0x30, 0x60, 0xFF, 0x0,//M
0x0, 0xFF, 0xFF, 0xDB, 0xDB, 0xC3, 0xC3, 0x0,//E
0x0, 0xFF, 0xFF, 0xD8, 0xD8, 0x77, 0x37, 0x0,//R
0x0, 0xFF, 0xFF, 0xD8, 0xD8, 0x77, 0x37, 0x0,//R
0x0, 0xF0, 0xF8, 0xF, 0xF, 0xF8, 0xF0, 0x0,//Y
0x0, 0x0, 0x0, 0x0, 0x0, 0x0,//Space
0x0, 0xC3, 0xE7, 0x7E, 0x7E, 0xE7, 0xC3, 0x0,//X
0x0, 0xFF, 0x60, 0x30, 0x30, 0x60, 0xFF, 0x0,//M
0x0, 0x7F, 0xFF, 0x8C, 0x8C, 0xFF, 0x7F, 0x0,//A
0x0, 0x26, 0x73, 0xDB, 0xDB, 0xDF, 0x66, 0x0,//S
0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0, 0x0,//Space
};
int pointer;
int column;
int pic;
void setup()
{
DDRC = B00011111; //Set pins A0 to A5 as Output.
DDRB = B00000011; //Set pins 8 and 9 as Output.
DDRD = B11111111; //Set pins 0 to 7 as Output.
}
void loop()
{
PORTC = B00000000; //All Columns OFF.
PORTB = B00000000; //All Columns OFF.
for(pointer=0; pointer < 110;pointer++){ //Load array pointer. 110 columns to limit array.
for (pic =0; pic < 5;pic++ ){//Control how many times the picture is repeated (you can change speed scrolling text)
for(column=0; column < 8;column++) // Count number of column
{
if (column<=5)
{
PORTB = B00000000;//Columns 7 and 6 as disabled
PORTC = B00000001 column;//set in sequence the columns 0 to 5 .
}
else
{
PORTC = B00000000;//
PORTB = B00000001 column-6;//set in sequence the columns 6 (Pin 8) and 7 (Pin 9).
}
PORTD = (text[pointer+column]);//Load column from the array to PORTD
delay(3);// Wait sometime for POV
PORTD = 0x00;
}
}
}
}
You can change the message on array text[].
Now you have a scrolling text message to say MERRY XMAS to everybody!
Reference:
http://en.wikipedia.org/wiki/Persistence_of_vision
http://www.arduino.cc/en/Reference/PortManipulation